Prompt
I asked my ChatGPT Slack Bot to analyze and explain some python code like below.
@ChatGPT Please analyze and explain the following python code.
# Program to display the Fibonacci sequence up to n-th term
nterms = int(input("How many terms? "))
# first two terms
n1, n2 = 0, 1
count = 0
# check if the number of terms is valid
if nterms <= 0:
print("Please enter a positive integer")
# if there is only one term, return n1
elif nterms == 1:
print("Fibonacci sequence upto",nterms,":")
print(n1)
# generate fibonacci sequence
else:
print("Fibonacci sequence:")
while count < nterms:
print(n1)
nth = n1 + n2
# update values
n1 = n2
n2 = nth
count += 1
Answer
This is a Python program that generates the Fibonacci sequence up to the n-th term.The program first prompts the user to enter the number of terms they want to generate. It then initializes the first two terms of the sequence, n1 and n2, to 0 and 1 respectively. It also initializes a counter variable, count, to 0.The program then checks if the number of terms entered by the user is valid. If it is less than or equal to 0, it prints an error message asking the user to enter a positive integer. If there is only one term, it simply prints the value of n1.If the number of terms is valid and greater than 1, the program enters a loop that generates the Fibonacci sequence. The loop continues until the count variable is equal to the number of terms entered by the user.Inside the loop, the program prints the current value of n1, which is the current term in the sequence. It then calculates the next term in the sequence, nth, by adding n1 and n2. It updates the values of n1 and n2 to be the previous two terms in the sequence, and increments the count variable.Finally, when the loop is finished, the program has generated the entire Fibonacci sequence up to the n-th term, and it prints the sequence to the console.
Prompt
@ChatGPT So what if I put 10 into the input. What's the execution result of the code?
Answer
If you enter 10 as the input, the program will generate the first 10 terms of the Fibonacci sequence and print them to the console. The output will look like this:
Fibonacci sequence:
0
1
1
2
3
5
8
13
21
34
The first two terms of the sequence are 0 and 1, and each subsequent term is the sum of the previous two terms. So the third term is 0 + 1 = 1, the fourth term is 1 + 1 = 2, the fifth term is 1 + 2 = 3, and so on, until the 10th term, which is 34.
Conclusion
Stunning and breath-taking.
Full Evidence
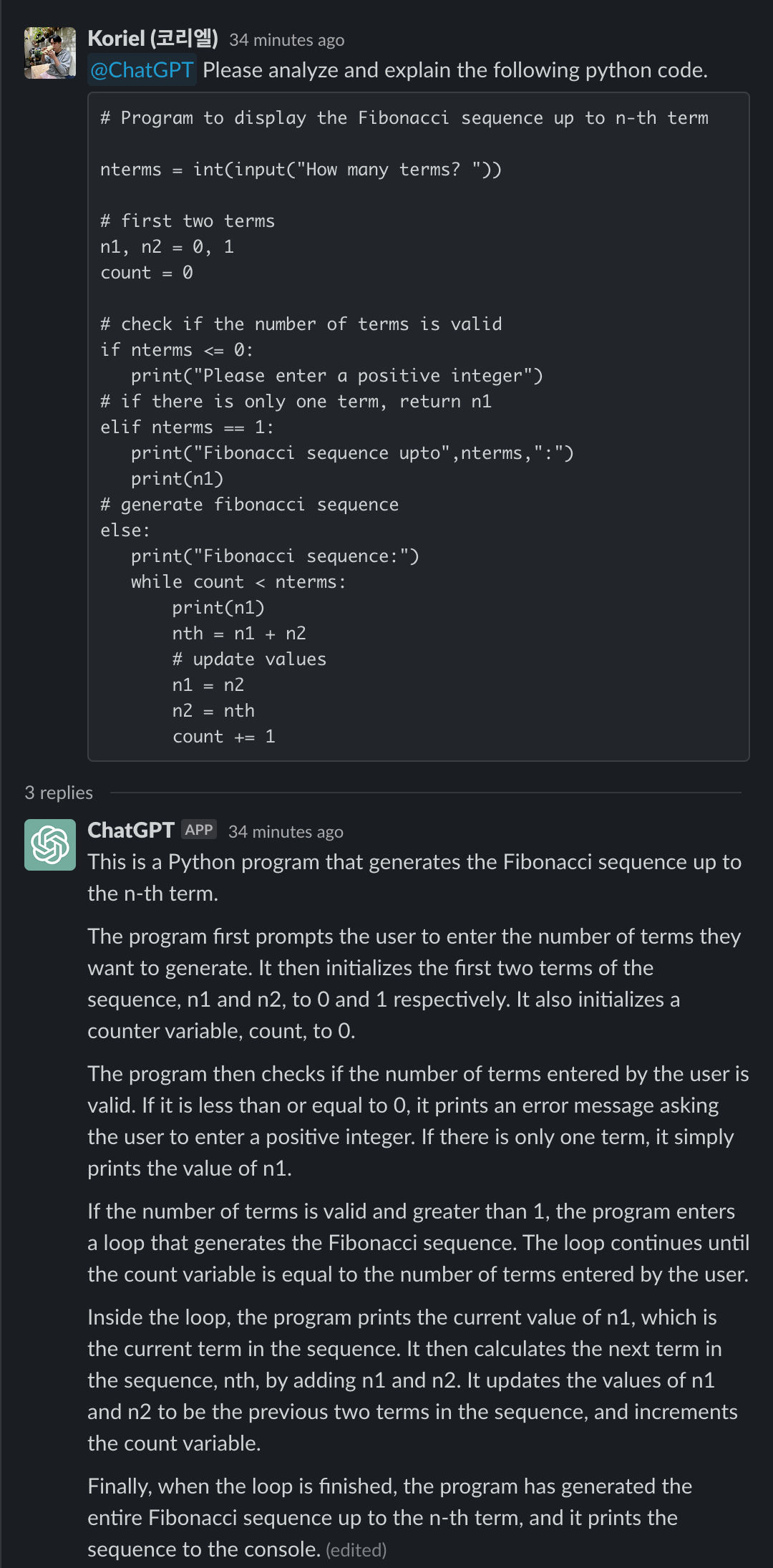
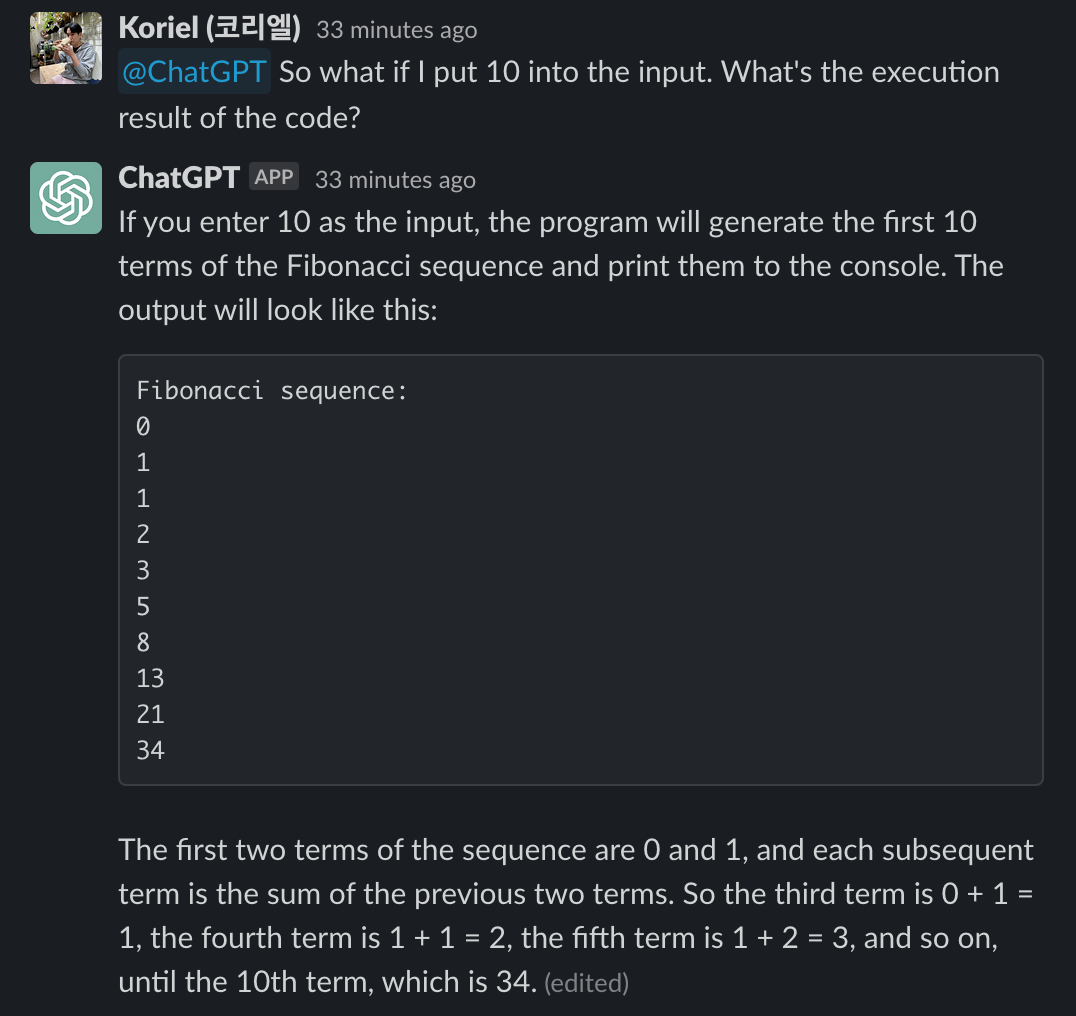